There are a few agile practices that I really love. Pair programming
is one of them.
We try to do it as much as possible at OmbuLabs. We
usually keep the sessions under two hours and try to follow a regular
schedule.
When we find ourselves blocked by a code problem, we use our daily scrum to
coordinate a pairing session. It’s quite a step up from rubberducking or using a cardboard programmer to find a solution
to a problem.
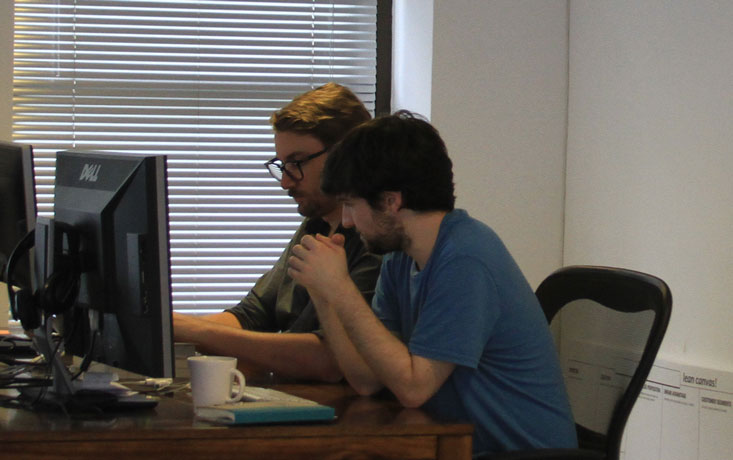
The Joys
As a Senior developer, I find that pairing sessions are great for coaching
Junior developers. I enjoy teaching them about best practices, design
patterns, frameworks, languages, code style,
XP, and
TDD.
From the point of view of a Junior developer, I believe it’s a
great opportunity to learn from someone who “has been there before”.
When you program with someone with more experience, you will often learn about
design patterns, elegant object-oriented solutions, tips and tricks.
Read more »